สร้าง RESTful API เบื้องต้นสำหรับ เพิ่ม/ลบ/แก้ไข ด้วย Node.js ร่วมกับ Express
วันที่: 7 เม.ย. 2565 23:00 น.
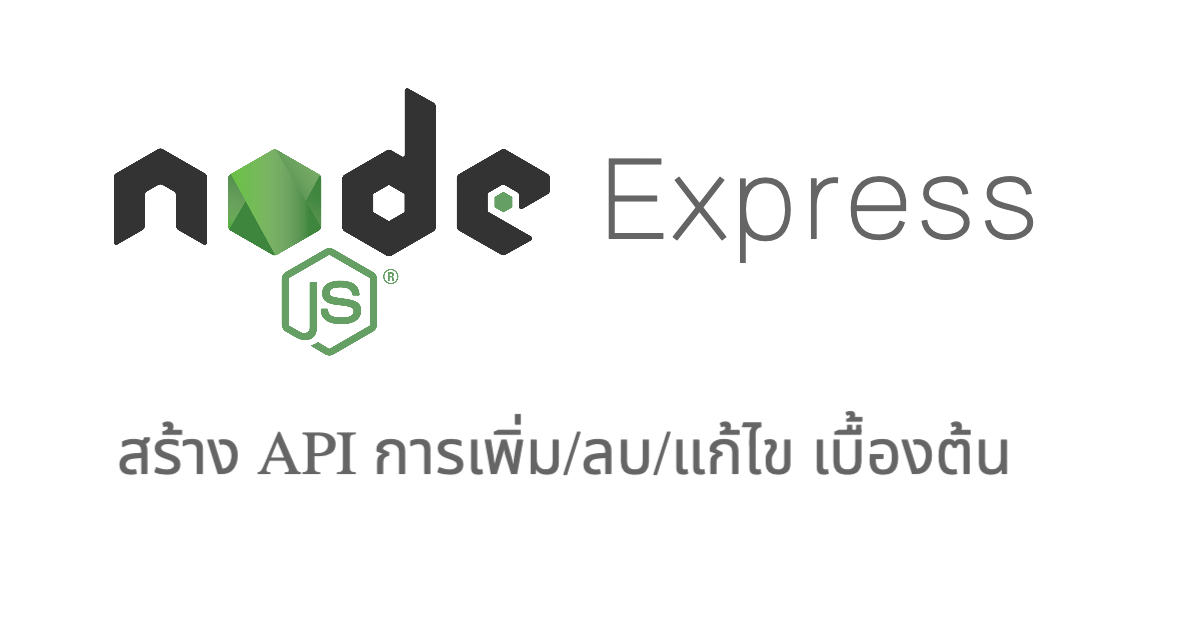
เมื่อได้อ่านเริ่มต้นสร้าง RESTful API ด้วย Node.js ร่วมกับ Expressกันแล้ว บทความนี้จะพาสร้าง RESTful API เบื้องต้นสำหรับการ เพิ่ม ลบ แก้ไข ข้อมูลด้วย Node.js ที่ทำงานร่วมกับ Express กัน จะเป็นพื้นฐานที่คาดว่าผู้อ่านจะทำความเข้าใจได้ง่าย ซึ่งจะใช้โค้ดต่อจากบทความที่แล้วนะครับ ใครยังไม่ได้อ่าน ไปอ่านได้เลย
ก่อนที่จะเริ่มโค้ดกัน เราจะติดตั้ง nodemon กันก่อนครับ เพื่อเวลาเราแก้ไขโค้ด ระบบเราจะอัพเดตให้อัตโนมัติเลย ด้วยโค้ดดังนี้
npm i nodemon
ออกแบบ API
- GET
/persons
สำหรับเรียกข้อมูลทั้งหมดในฐานข้อมูล - POST
/person
สำหรับเพิ่มข้อมูล - GET
/person/:id
สำหรับเรียกข้อมูลตาม id ที่กำหนด - PUT
/person/:id
สำหรับแก้ไขข้อมูลตาม id ที่กำหนด - DELETE
/person/:id
สำหรับลบข้อมูลตาม id ที่กำหนด
ในกรณีนี้ เราจะใช้ postman ในการเรียกทดสอบ API ครับ ใครยังไม่มีก็ติดตั้งให้เรียบร้อย คือใครมีตัวอื่นก็สามารถใช้ได้เช่นกัน
จำลองฐานข้อมูลด้วย JSON ไฟล์
ให้สร้างไฟล์ mydb.json ขึ้นมาในโปรเจคของเรา โดยมีโค้ดดังนี้ (ตรง name ก็แล้วแต่จะตั้งได้นะ ส่วนกรณีนี้ผมเอามาจาก https://randomuser.me)
[
{
"id": 1,
"name": "Beverley Lawson"
},
{
"id": 2,
"name": "Ramon Gregory"
},
{
"id": 3,
"name": "Brad Rogers"
}
]
เริ่มสร้าง API กันเลย
ก่อนอื่นขอตรวจสอบกันก่อนว่าโค้ดตั้งต้น เท่ากันแล้วหรือยังนะ ถ้าใครยังไม่เท่า ก็ให้เริ่มจากโค้ดด้านล่างนี้ก่อนเลย จะได้เท่ากันกับบทความนี้ครับ
const express = require("express");
const app = express();
const port = process.env.PORT || 3000;
app.get("/", (req, res) => {
res.send("Welcome!");
});
app.listen(port, () => {
console.log("starting server at port " + port);
});
1. สร้าง API สำหรับเรียกข้อมูลทั้งหมดในฐานข้อมูล ด้วยโค้ดดังนี้
// get all person
app.get('/persons', (req, res) => {
res.json(persons);
});
ผลการทดสอบ
2. สร้าง API สำหรับเพิ่มข้อมูล ด้วยโค้ดดังนี้
// add new person
app.post('/person', (req, res) => {
persons.push(req.body);
let json = req.body;
res.send(`add '${json.name}' successfully!`);
});
ผลการทดสอบ
3. สร้าง API สำหรับเรียกข้อมูลตาม id ที่กำหนด ด้วยโค้ดดังนี้
// get person by id
app.get('/person/:id', (req, res) => {
const person = persons.find(p => p.id === Number(req.params.id));
res.json(person);
});
ผลการทดสอบ
4. สร้าง API สำหรับแก้ไขข้อมูลตาม id ที่กำหนด ด้วยโค้ดดังนี้
// update person by id
app.put('/person/:id', (req, res) => {
const index = persons.findIndex(p => p.id === Number(req.params.id));
res.send(`update person id: '${persons[index].id}' successfully!`);
});
ผลการทดสอบ
5. สร้าง API สำหรับลบข้อมูลตาม id ที่กำหนด ด้วยโค้ดดังนี้
// delete person by id
app.delete('/person/:id', (req, res) => {
const index = persons.findIndex(p => p.id === Number(req.params.id));
res.send(`delete '${persons[index].name}' successfully!`);
});
ผลการทดสอบ
สรุปกันนิดนึง
โค้ดทั้งหมดในไฟล์ server.js จะมีหน้าตาแบบนี้นะครับ
const express = require("express");
const app = express();
const port = process.env.PORT || 3000;
const persons = require('./mydb');
app.use(express.json());
app.use(express.urlencoded({ extended: true }));
app.get("/", (req, res) => {
res.send("Welcome!");
});
// get all person
app.get('/persons', (req, res) => {
res.json(persons);
});
// add new person
app.post('/person', (req, res) => {
persons.push(req.body);
let json = req.body;
res.send(`add '${json.name}' successfully!`);
});
// get person by id
app.get('/person/:id', (req, res) => {
const person = persons.find(p => p.id === Number(req.params.id));
res.json(person);
});
// update person by id
app.put('/person/:id', (req, res) => {
const index = persons.findIndex(p => p.id === Number(req.params.id));
res.send(`update person id: '${persons[index].id}' successfully!`);
});
// delete person by id
app.delete('/person/:id', (req, res) => {
const index = persons.findIndex(p => p.id === Number(req.params.id));
res.send(`delete '${persons[index].name}' successfully!`);
});
app.listen(port, () => {
console.log("starting server at port " + port);
});
นี่เป็นเพียงจุดเริ่มต้นที่คาดว่าจะเข้าใจง่ายที่สุดแล้วครับ หวังว่าจะเป็นประโยชน์และสามารถนำไปต่อยอดกับงานอื่น ๆ ได้ครับ
เรื่องอื่น ๆ ที่เกี่ยวข้อง
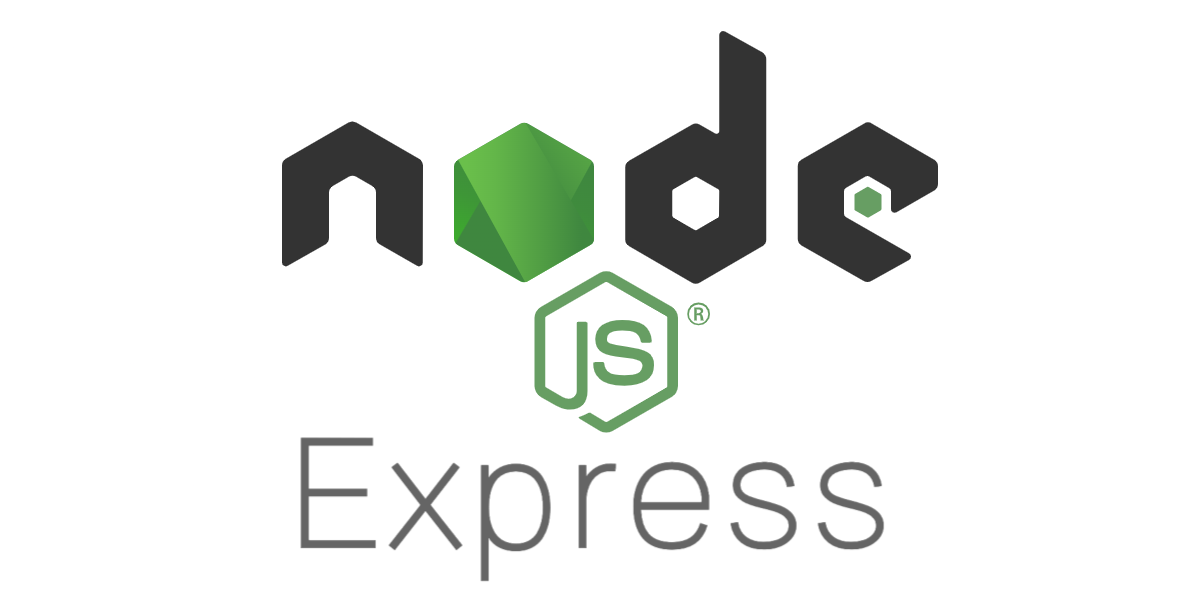
เริ่มต้นสร้าง RESTful API ด้วย Node.js ร่วมกับ Express
เมื่อวันที่: 6 เม.ย. 2565 22:57 น.
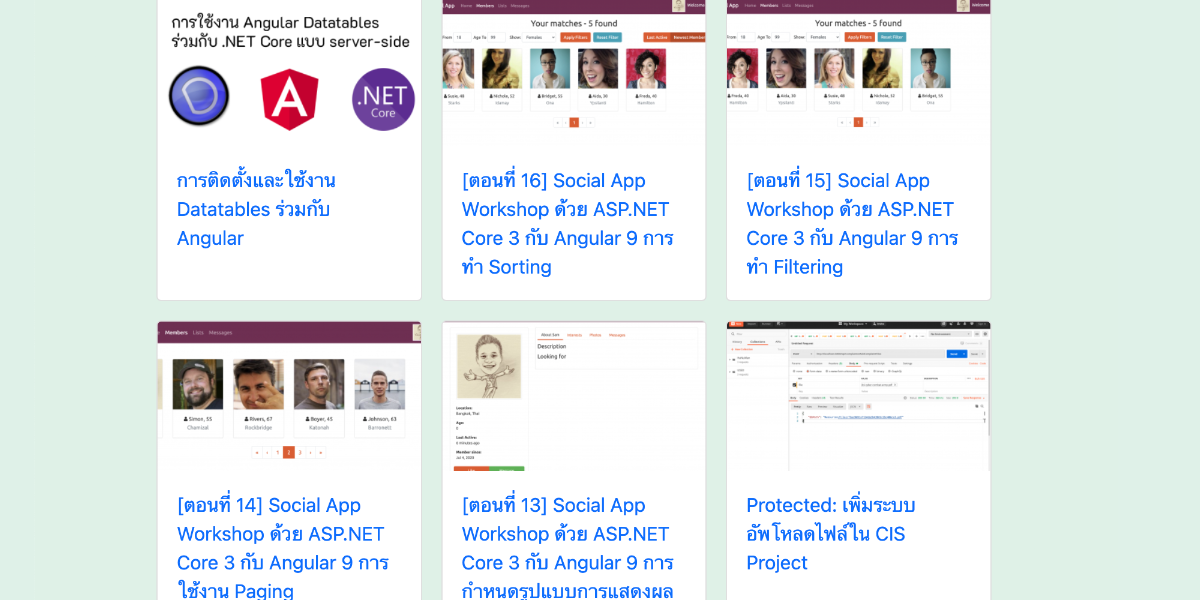
Web Scraping ดึงข้อมูลจากเว็บไซต์ด้วยภาษา PHP
เมื่อวันที่: 5 พ.ค. 2565 22:06 น.
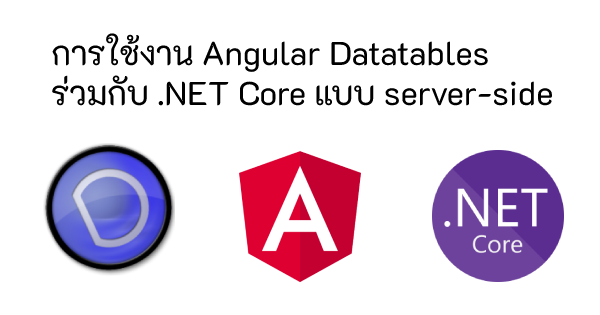
การใช้งาน Angular ร่วมกับ Datatables เรียกใช้ข้อมูลจาก .NET Core แบบ Server-side
เมื่อวันที่: 18 ก.พ. 2565 09:48 น.
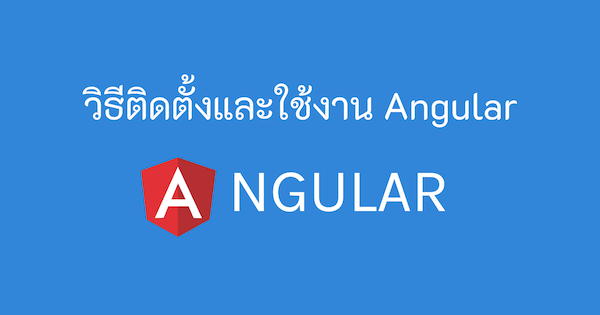
เมื่อวันที่: 16 ก.พ. 2565 16:13 น.
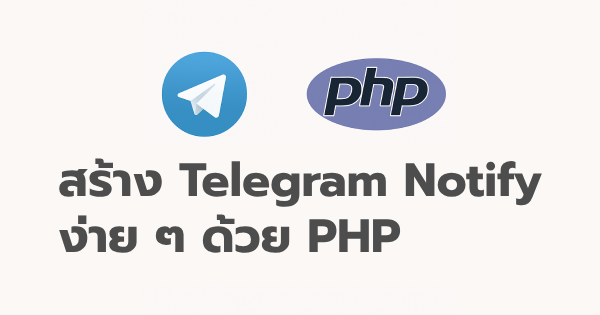
โค้ด PHP สำหรับสร้าง Telegram Notify อย่างง่าย
เมื่อวันที่: 2 เม.ย. 2568 12:05 น.
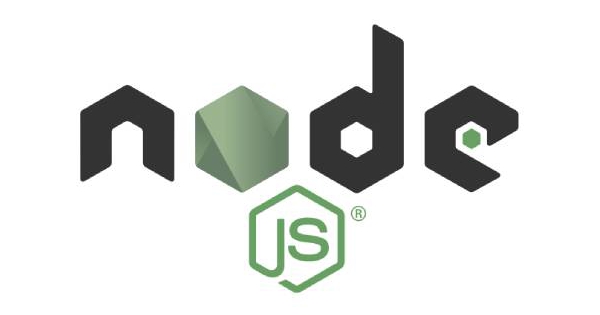
วิธีติดตั้ง Node.js บน Macbook (macOS)
เมื่อวันที่: 13 ก.พ. 2565 23:13 น.